Question
Design and implement a simple calculator program that performs addition, subtraction, multiplication, and division on two numbers given by the user. In this assignment, all
Design and implement a simple calculator program that performs addition, subtraction, multiplication, and division on two numbers given by the user. In this assignment, all output must match this specification; do not provide any additional questions or any customization.
You will first prompt the user with a menu:
Welcome to the Simple Calculator menu.
1. Addition
2. Subtraction
3. Multiplication
4. Division
5. Exit
Which operation would you like to perform (1-5): _
If the user enters in 5, output the following and exit the program:
Thank you for using the Simple Calculator. Goodbye.
If the user enters in 1-4, prompt the user for two numbers, perform the appropriate operation, output the answer, and prompt the user with the menu again. Repeat these steps until the user selects 5 to exit the program.
You have selected Multiplication.
Enter the value for x: _
Enter the value for y: _
x + y = #
Guidelines:
You must have separate functions for Addition, Subtraction, Multiplication, and Division.
You are not permitted to use the * or / operators.
You must use if-elif-else, while loops, for loops, and functions in your code.
The user can input integers and floating point numbers.
Your calculations should return floating point numbers.
Submit your script as calculator.py
Here is my code but I am instructed to not use operators * or / and cannot figure how to. Can you help?
#Functions add, subtract, multiply and divide
# adds two numbers
def add(x, y):
return x + y
# subtracts two numbers
def subtract(x, y):
return x - y
# multiplies two numbers
def multiply(x, y):
return x * y
# divides two numbers
def divide(x, y):
return x / y
print("Welcome to the Simple Calculator menu")
print("1.Addition")
print("2.Subtraction")
print("3.Multiplication")
print("4.Division")
print("5.Exit")
# input from the keyboard
choice = input("Which operation would you like to perform (1-5):")
if choice == '5':
print('Thank you for using the Simple Calculator. Goodbye')
quit()
num1 = int(input("Enter first number: "))
num2 = int(input("Enter second number: "))
if choice == '1':
print(num1,"+",num2,"=", add(num1,num2))
elif choice == '2':
print(num1,"-",num2,"=", subtract(num1,num2))
elif choice == '3':
print(num1,"*",num2,"=", multiply(num1,num2))
elif choice == '4':
print(num1,"/",num2,"=", divide(num1,num2))
else:
print("Invalid input")
Step by Step Solution
There are 3 Steps involved in it
Step: 1
CODE adds two numbers def addx y return x y subtracts two numbers def subtractx y return x y multiplies two numbers def multiplyx y result0 logic to calculate the multiplication of two numbers for i i...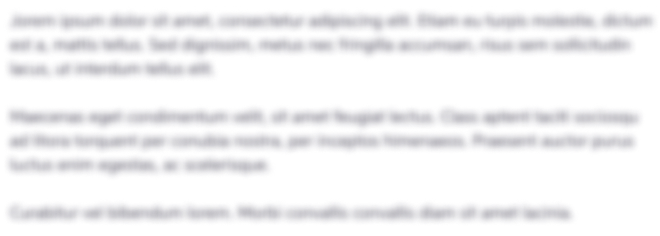
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started