Question
I have to classes and I want to add or implement polymorphism and inheritance in my code as well as to apply exception to all
I have to classes and I want to add or implement polymorphism and inheritance in my code as well as to apply exception to all my input validations. below here is the code:
import java.util.Scanner; public class ClientCarolino {
public static void main(String[] args) { CreditCarolino[] credit = new CreditCarolino[100]; Scanner input = new Scanner(System.in); int choice;
do { System.out.println("Java Credit Main Menu"); System.out.println("[1] New Credit Account"); System.out.println("[2] Credit Inquiry"); System.out.println("[3] Purchases"); System.out.println("[4] Payment"); System.out.println("[5] Close Credit Account"); System.out.println("[7] Exit"); System.out.print("Enter choice: "); choice = input.nextInt();
switch (choice) { case 1: for (int i = 0; i < credit.length; i++) { if (credit[i] == null) { credit[i] = new CreditCarolino(); credit[i].createAccount(); break; } } break; case 2: System.out.print("Enter credit account number: "); int num = input.nextInt(); for (int i = 0; i < credit.length; i++) { if (credit[i] != null && credit[i].getAccountNumber() == num) { credit[i].inquiry(); break; } } break; case 3: System.out.print("Enter credit account number: "); num = input.nextInt(); for (int i = 0; i < credit.length; i++) { if (credit[i] != null && credit[i].getAccountNumber() == num) { credit[i].purchase(); break; } } break; case 4: System.out.print("Enter credit account number: "); num = input.nextInt(); for (int i = 0; i < credit.length; i++) { if (credit[i] != null && credit[i].getAccountNumber() == num) { credit[i].payment(); break; } } break; case 5: System.out.print("Enter credit account number: "); num = input.nextInt(); for (int i = 0; i < credit.length; i++) { if (credit[i] != null && credit[i].getAccountNumber() == num) { credit[i].closeAccount(); break; } } break; } } while (choice != 7); } }
import java.util.Scanner; public class CreditCarolino { private int accountNumber; private String accountName; private double creditLimit; private double creditBalance;
public void createAccount() { Scanner input = new Scanner(System.in); System.out.print("Enter client name: "); accountName = input.nextLine(); System.out.print("Enter annual income: "); int income = input.nextInt();
if (income >= 200000 && income <= 300000) { creditLimit = 30000; } else if (income >= 300000 && income <= 500000) { creditLimit = 50000; } else if (income >= 500000) { creditLimit = 100000; } else { System.out.println("Credit limit not qualified."); return; }
accountNumber = (int) (Math.random() * 9000) + 1000; System.out.println("Credit account number: " + accountNumber); System.out.println("Credit account name: " + accountName); System.out.println("Credit limit: " + creditLimit); System.out.println("Credit balance: " + creditBalance); }
public void inquiry() { System.out.println("Credit account number: " + accountNumber); System.out.println("Credit account name: " + accountName); System.out.println("Credit limit: " + creditLimit); System.out.println("Credit balance: " + creditBalance); }
public void purchase() { Scanner input = new Scanner(System.in); System.out.print("Enter amount of purchases: "); double purchase = input.nextDouble();
if (purchase < 1) { System.out.println("Invalid amount."); return; }
if (purchase > creditLimit) { System.out.println("Amount exceeded credit limit."); return; }
creditBalance += purchase; creditBalance += creditBalance * 0.03; creditLimit -= creditBalance; System.out.println("Credit balance: " + creditBalance); System.out.println("Allowable purchase amount: " + creditLimit); }
public void payment() { Scanner input = new Scanner(System.in); System.out.print("Enter amount of payment: "); double payment = input.nextDouble();
if (payment < 1) { System.out.println("Invalid amount."); return; }
if (payment > creditBalance) { System.out.println("Amount exceeded credit balance."); return; }
creditBalance -= payment; System.out.println("Credit balance: " + creditBalance); }
public void closeAccount() { Scanner input = new Scanner(System.in); System.out.print("Are you sure you want to close account? [Y/N]: "); String confirm = input.next();
if (confirm.equalsIgnoreCase("Y")) { if (creditBalance > 0) { System.out.println("Credit balance not yet fully paid."); return; } accountNumber = 0; accountName = ""; creditLimit = 0; creditBalance = 0; System.out.println("Account successfully closed."); } }
public int getAccountNumber() { return accountNumber; } }
Kindly help regarding this matter. Thank you
Step by Step Solution
There are 3 Steps involved in it
Step: 1
Answer Heres how you can modify your code import javautilScanner Superclass Account class Account protected int accountNumber protected String account...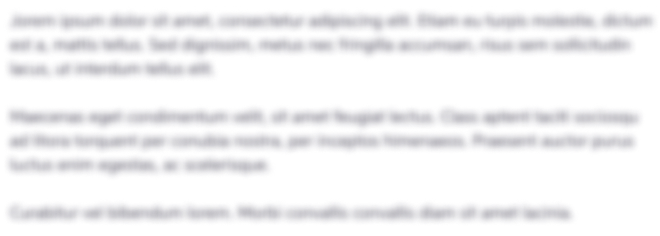
Get Instant Access with AI-Powered Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started