Question
Please make sure to test the program with different courses and grades no error to see the algorithm works fine. People at College Objective
Please make sure to test the program with different courses and grades no error to see the algorithm works fine.
People at College
- Objective
In this project, you'll create several classes using inheritance and polymorphism. This will require you to create abstract method and class. There won't be any exciting UI here, just some output of array data.
- Structure
- There are two types of college people: Students and Teachers.
- All people have an ID, Name, and Email.
- All IDs have validation rules, but rules differ:
- Students - 9 digits;
- Teachers - 6 alphanumeric.
- For Students, we want to maintain a list of courses taken (up to 30 courses) and associated grades; we want to obtain overall grade averages as well.
- For Teachers, we record courses they have taught (up to 50); we want a way to add courses to that list.
Person |
-id : String |
-name : String |
-email : String |
Constructors |
+Person(id : String, name: String) +Person(id : String, name: String, email : String ) |
Accessors |
+getId() : String |
+getName() : String |
+getEmail() : String |
Mutators +setId(id : String) +setName(name: String) +setEmail(email : String) |
Other Methods |
+isValidId(id : String) : Boolean |
+toString() : String |
+test() |
** isValidId() method should be an abstract.
An UML class diagram
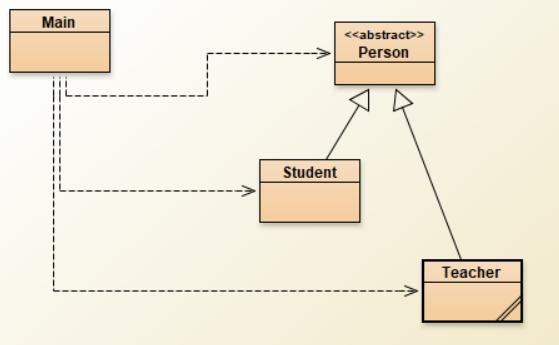
Students |
-courseTaken : String[30] |
-courseGrades : Double[30] |
-nextCourseIndex : Integer |
Constructor |
+ Students (id : String, name: String, email : String ) |
Methods |
+isValidIdId(id : String) : Boolean |
+courseCompletion(courseName : String, courseGrade : Double) |
+getAverageGrade() : Double |
+toString() : String |
+test() |
Teachers |
-coursesTaught: String[50] |
-nextCourseIndex : Integer |
Constructor |
+ Students (id : String, name: String, email : String ) |
Methods |
+isValidIdId(id : String) : Boolean |
+addCourseTaught (courseName : String) |
+toString() : String |
+test() |
- Code Implementation
Create main class which creates a new instance of the Students and Teachers classes and displays all information. See examples of output.
- Testing
- Write test methods for each of your methods.
- Test with different courses and grades to make sure your algorithms are correct.
- Manual inspection will be necessary; the debugger is your friend and will be very useful here.
Examples of Output
Id = 123456789
Name = Bobby Brown
Email = some email
Current grade average is 3.075
Student has completed 4 course (s) so far:
- History of Western Civilizations (1.7)
- Algebra II (3.9)
- Geometry I (3.7)
- History of Western Civilizations (3.0)
Id = ss9482
Name = Samantha Smith
Email = some email
Has taught 2 course (s) :
- Algebra II
- Intro to programming
Id = 987654321
Name = Chrissy Caldwell
Email = some email
Current grade average is 4.0
Student has completed 1 couse (s) so far:
-Sociology I (4.0)
Main Student < > Person Teacher
Step by Step Solution
There are 3 Steps involved in it
Step: 1
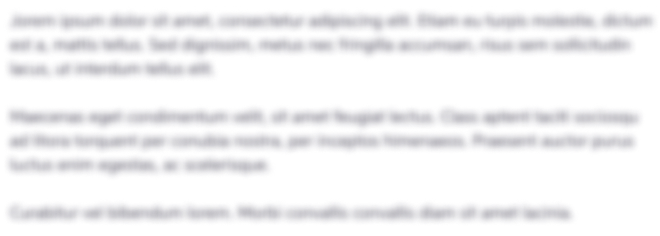
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started