Implement these four classes using Python. Below is a brief description of those classes: 1. Location...
Fantastic news! We've Found the answer you've been seeking!
Question:
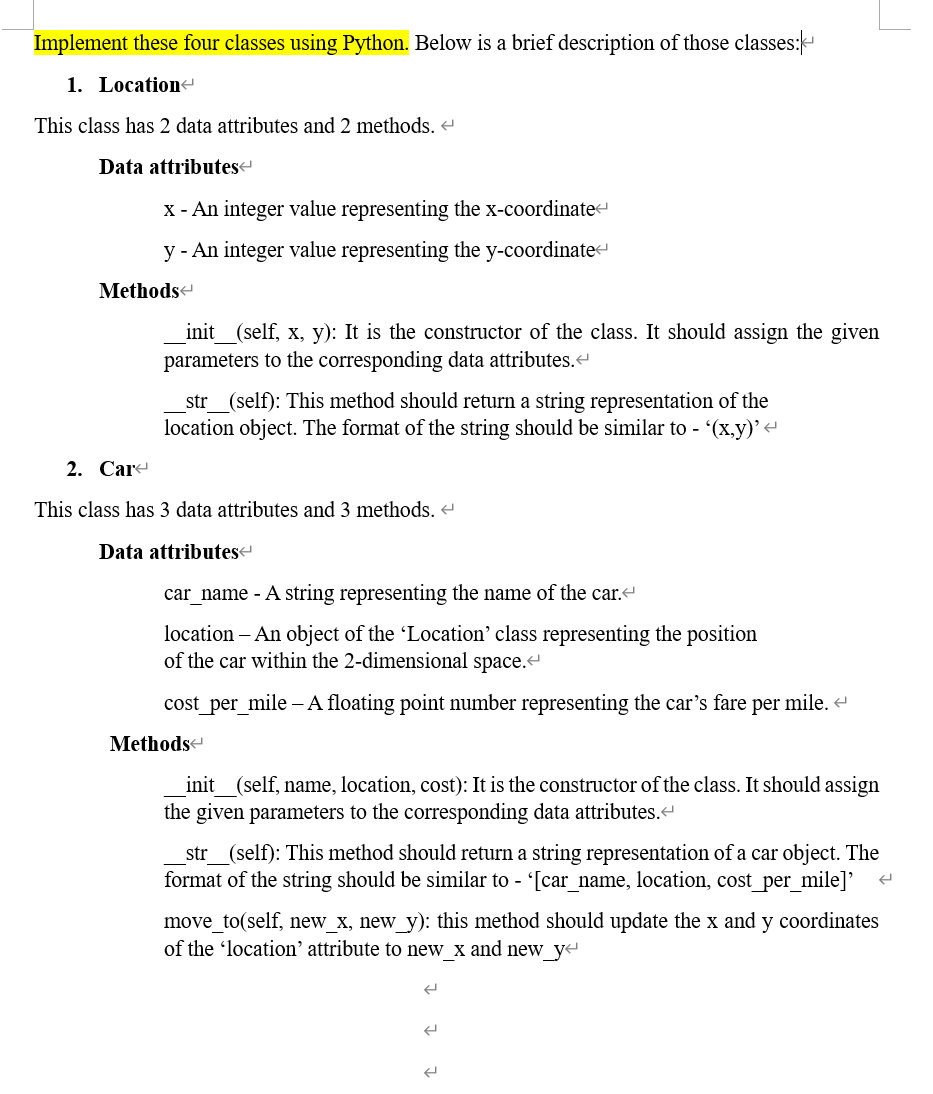
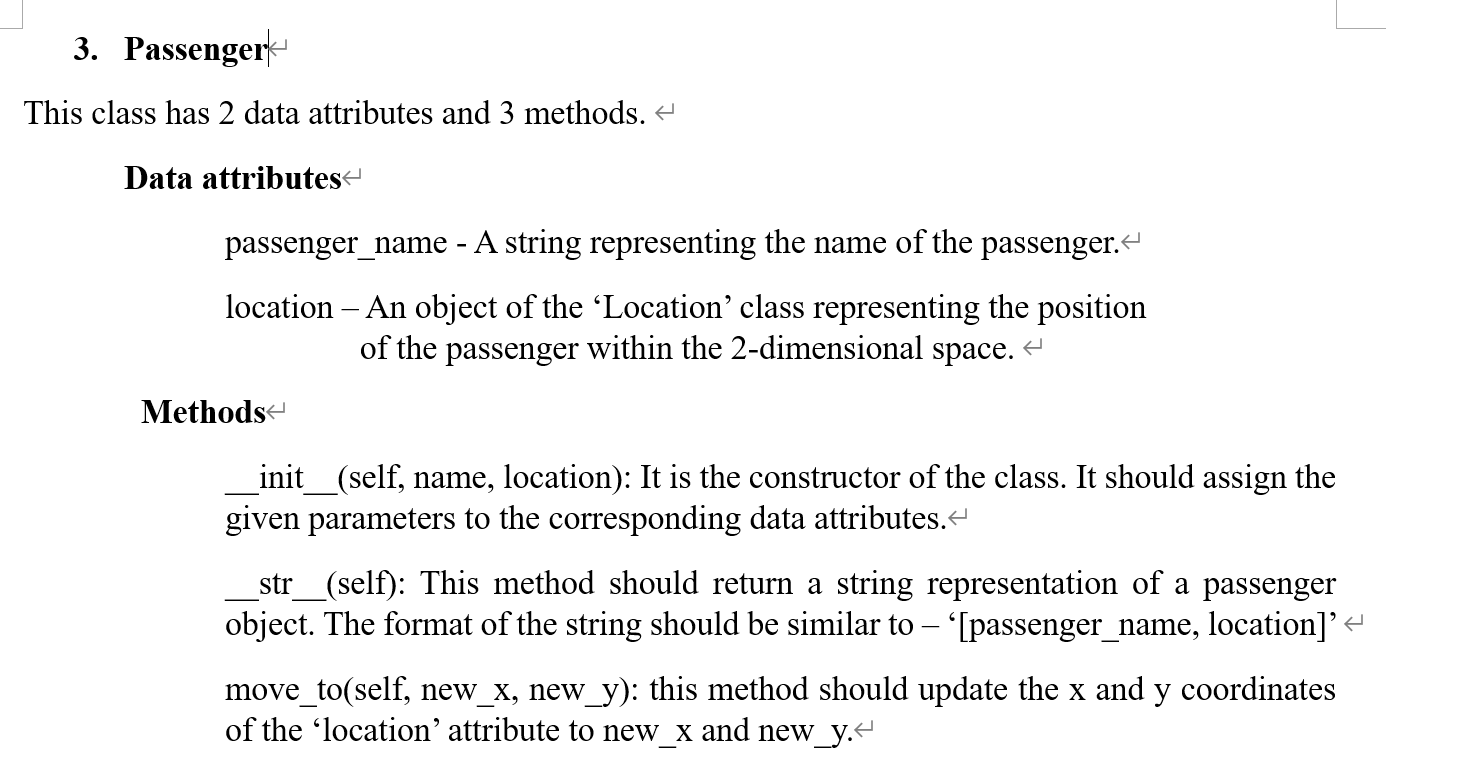
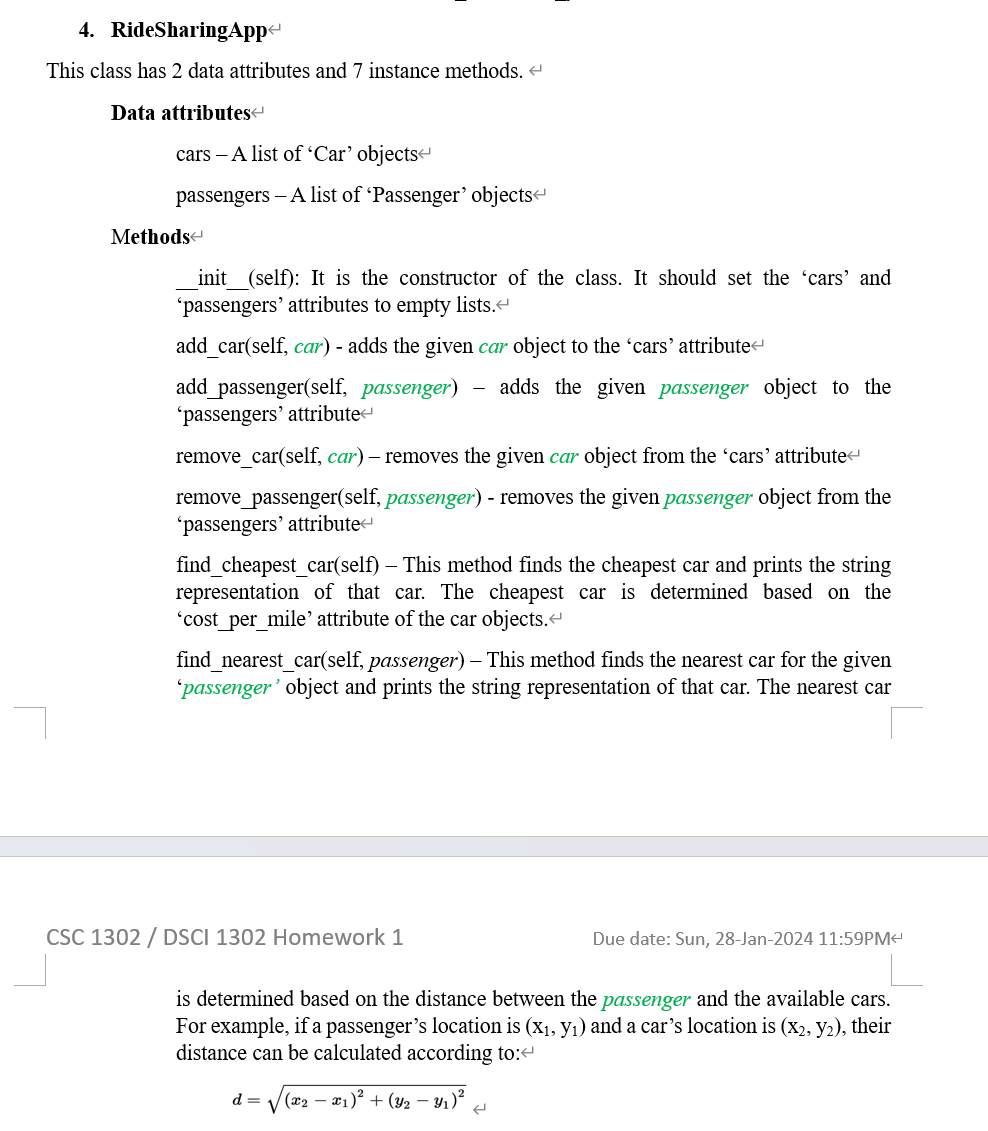
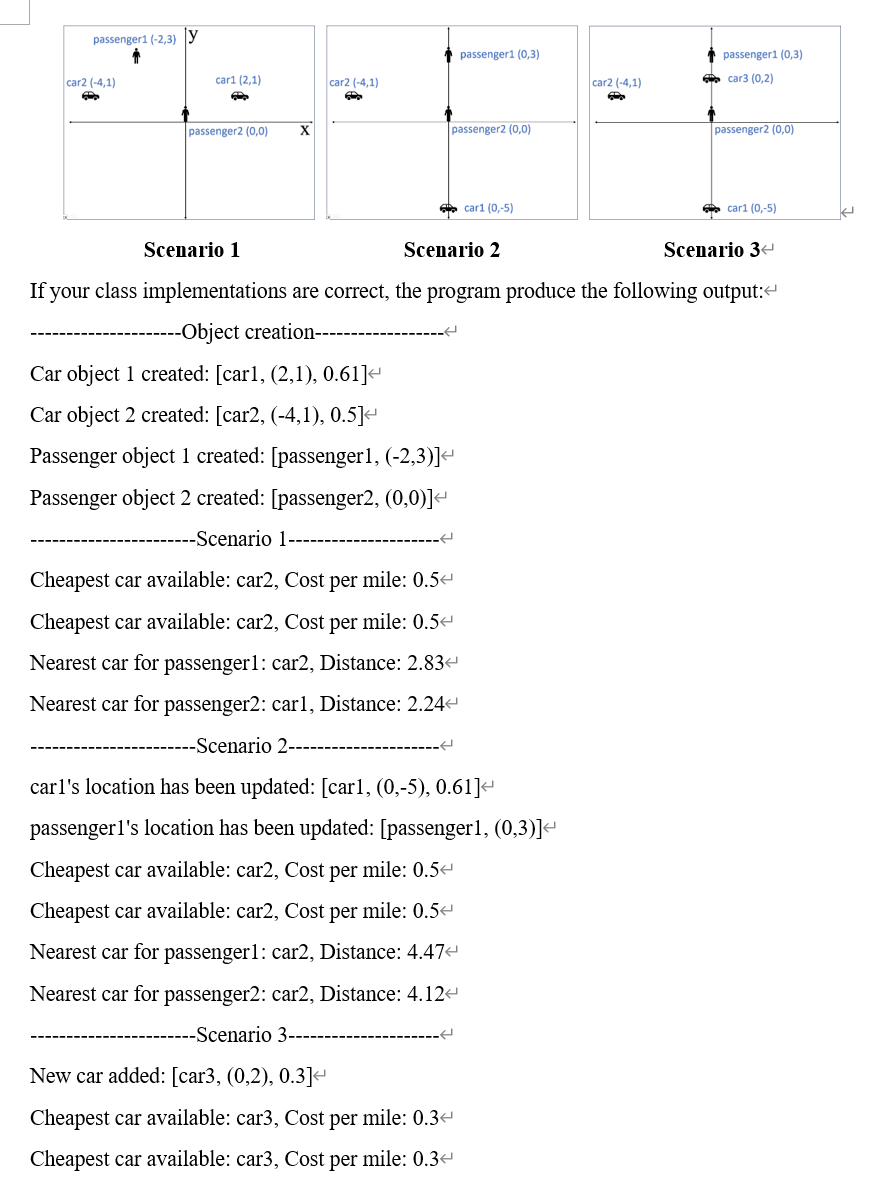
Transcribed Image Text:
Implement these four classes using Python. Below is a brief description of those classes: 1. Location < This class has 2 data attributes and 2 methods. < Data attributes < x - An integer value representing the x-coordinate < y - An integer value representing the y-coordinate < Methods 2. Car _init__(self, x, y): It is the constructor of the class. It should assign the given parameters to the corresponding data attributes. _str_(self): This method should return a string representation of the location object. The format of the string should be similar to - '(x,y)' < This class has 3 data attributes and 3 methods. < Data attributes < car_name - A string representing the name of the car. < location - An object of the 'Location' class representing the position of the car within the 2-dimensional space. < cost per mile - A floating point number representing the car's fare per mile. Methods _init__(self, name, location, cost): It is the constructor of the class. It should assign the given parameters to the corresponding data attributes. < _str_(self): This method should return a string representation of a car object. The format of the string should be similar to - [car_name, location, cost_per_mile]' move_to(self, new_x, new_y): this method should update the x and y coordinates of the 'location' attribute to new_x and new_y 3. Passenger This class has 2 data attributes and 3 methods. < Data attributes < passenger_name - A string representing the name of the passenger. < location An object of the 'Location' class representing the position of the passenger within the 2-dimensional space. < Methods _init__(self, name, location): It is the constructor of the class. It should assign the given parameters to the corresponding data attributes. < _str_(self): This method should return a string representation of a passenger object. The format of the string should be similar to - '[passenger_name, location]' < move_to(self, new_x, new_y): this method should update the x and y coordinates of the 'location' attribute to new_x and new_y. 4. RideSharingApp < This class has 2 data attributes and 7 instance methods. < Data attributes < cars - A list of 'Car' objects < passengers A list of 'Passenger' objects < Methods _init_(self): It is the constructor of the class. It should set the 'cars' and 'passengers' attributes to empty lists. < add_car(self, car) - adds the given car object to the 'cars' attribute < add passenger(self, passenger) 'passengers' attribute < - adds the given passenger object to the remove_car(self, car) - removes the given car object from the 'cars' attribute < remove passenger(self, passenger) - removes the given passenger object from the 'passengers' attribute < find_cheapest_car(self) This method finds the cheapest car and prints the string representation of that car. The cheapest car is determined based on the 'cost_per_mile' attribute of the car objects. < find_nearest_car(self, passenger) This method finds the nearest car for the given 'passenger' object and prints the string representation of that car. The nearest car CSC 1302/DSCI 1302 Homework 1 Due date: Sun, 28-Jan-2024 11:59PM < is determined based on the distance between the passenger and the available cars. For example, if a passenger's location is (X1, y) and a car's location is (X2, y2), their distance can be calculated according to: < d = (x2-2 x1) +(32-41) passenger1 (-2,3) car2 (-4,1) carl (2,1) car2 (-4,1) passenger2 (0,0) passenger1 (0,3) passenger2 (0,0) car1 (0,-5) car2 (-4,1) passenger1 (0,3) car3 (0,2) passenger2 (0,0) car1 (0,-5) Scenario 1 Scenario 2 Scenario 3+ If your class implementations are correct, the program produce the following output: < ---Object creation------- Car object 1 created: [car1, (2,1), 0.61] < Car object 2 created: [car2, (-4,1), 0.5] < Passenger object 1 created: [passenger1, (-2,3)] < Passenger object 2 created: [passenger2, (0,0)] < Scenario 1 Cheapest car available: car2, Cost per mile: 0.5 < Cheapest car available: car2, Cost per mile: 0.5 < Nearest car for passenger1: car2, Distance: 2.83 < Nearest car for passenger2: carl, Distance: 2.24 < -Scenario 2---- carl's location has been updated: [car1, (0,-5), 0.61] < passengerl's location has been updated: [passenger1, (0,3)] < Cheapest car available: car2, Cost per mile: 0.5 < Cheapest car available: car2, Cost per mile: 0.5 < Nearest car for passenger1: car2, Distance: 4.47 < Nearest car for passenger2: car2, Distance: 4.12 < -Scenario 3--- New car added: [car3, (0,2), 0.3] < Cheapest car available: car3, Cost per mile: 0.3 < Cheapest car available: car3, Cost per mile: 0.3 Implement these four classes using Python. Below is a brief description of those classes: 1. Location < This class has 2 data attributes and 2 methods. < Data attributes < x - An integer value representing the x-coordinate < y - An integer value representing the y-coordinate < Methods 2. Car _init__(self, x, y): It is the constructor of the class. It should assign the given parameters to the corresponding data attributes. _str_(self): This method should return a string representation of the location object. The format of the string should be similar to - '(x,y)' < This class has 3 data attributes and 3 methods. < Data attributes < car_name - A string representing the name of the car. < location - An object of the 'Location' class representing the position of the car within the 2-dimensional space. < cost per mile - A floating point number representing the car's fare per mile. Methods _init__(self, name, location, cost): It is the constructor of the class. It should assign the given parameters to the corresponding data attributes. < _str_(self): This method should return a string representation of a car object. The format of the string should be similar to - [car_name, location, cost_per_mile]' move_to(self, new_x, new_y): this method should update the x and y coordinates of the 'location' attribute to new_x and new_y 3. Passenger This class has 2 data attributes and 3 methods. < Data attributes < passenger_name - A string representing the name of the passenger. < location An object of the 'Location' class representing the position of the passenger within the 2-dimensional space. < Methods _init__(self, name, location): It is the constructor of the class. It should assign the given parameters to the corresponding data attributes. < _str_(self): This method should return a string representation of a passenger object. The format of the string should be similar to - '[passenger_name, location]' < move_to(self, new_x, new_y): this method should update the x and y coordinates of the 'location' attribute to new_x and new_y. 4. RideSharingApp < This class has 2 data attributes and 7 instance methods. < Data attributes < cars - A list of 'Car' objects < passengers A list of 'Passenger' objects < Methods _init_(self): It is the constructor of the class. It should set the 'cars' and 'passengers' attributes to empty lists. < add_car(self, car) - adds the given car object to the 'cars' attribute < add passenger(self, passenger) 'passengers' attribute < - adds the given passenger object to the remove_car(self, car) - removes the given car object from the 'cars' attribute < remove passenger(self, passenger) - removes the given passenger object from the 'passengers' attribute < find_cheapest_car(self) This method finds the cheapest car and prints the string representation of that car. The cheapest car is determined based on the 'cost_per_mile' attribute of the car objects. < find_nearest_car(self, passenger) This method finds the nearest car for the given 'passenger' object and prints the string representation of that car. The nearest car CSC 1302/DSCI 1302 Homework 1 Due date: Sun, 28-Jan-2024 11:59PM < is determined based on the distance between the passenger and the available cars. For example, if a passenger's location is (X1, y) and a car's location is (X2, y2), their distance can be calculated according to: < d = (x2-2 x1) +(32-41) passenger1 (-2,3) car2 (-4,1) carl (2,1) car2 (-4,1) passenger2 (0,0) passenger1 (0,3) passenger2 (0,0) car1 (0,-5) car2 (-4,1) passenger1 (0,3) car3 (0,2) passenger2 (0,0) car1 (0,-5) Scenario 1 Scenario 2 Scenario 3+ If your class implementations are correct, the program produce the following output: < ---Object creation------- Car object 1 created: [car1, (2,1), 0.61] < Car object 2 created: [car2, (-4,1), 0.5] < Passenger object 1 created: [passenger1, (-2,3)] < Passenger object 2 created: [passenger2, (0,0)] < Scenario 1 Cheapest car available: car2, Cost per mile: 0.5 < Cheapest car available: car2, Cost per mile: 0.5 < Nearest car for passenger1: car2, Distance: 2.83 < Nearest car for passenger2: carl, Distance: 2.24 < -Scenario 2---- carl's location has been updated: [car1, (0,-5), 0.61] < passengerl's location has been updated: [passenger1, (0,3)] < Cheapest car available: car2, Cost per mile: 0.5 < Cheapest car available: car2, Cost per mile: 0.5 < Nearest car for passenger1: car2, Distance: 4.47 < Nearest car for passenger2: car2, Distance: 4.12 < -Scenario 3--- New car added: [car3, (0,2), 0.3] < Cheapest car available: car3, Cost per mile: 0.3 < Cheapest car available: car3, Cost per mile: 0.3
Expert Answer:
Related Book For
Posted Date:
Students also viewed these algorithms questions
-
Emery Company sells small machine parts to heavy equipment manufacturers for an average price of $1.30 per part. There are two types of customers: those who place small, frequent orders and those who...
-
Predictive text entry systems are familiar on touch screens and mobile phones. This question asks you to consider how the same principles might be used in a programming editor for creating Java code....
-
CANMNMM January of this year. (a) Each item will be held in a record. Describe all the data structures that must refer to these records to implement the required functionality. Describe all the...
-
Its estimated that the average corporate user sends and receives some 112 e-mails daily.78 Thats about 14 e-mails per hour, and even if half of those dont require a lot of time and concentration,...
-
The following are independent events: a. Changed from the FIFO to the LIFO inventory cost flow assumption. b. Wrote off patent due to the introduction of a competing product. c. Changed accounting...
-
How long (in years) would $600 have to be invested at 8%, compounded continuously, to amount to $970?
-
Jam Manufacturing Inc. has beginning work in process \($27,200\), direct materials used \($240,000\), di- rect labor \($200,000\), total manufacturing overhead \($150,000\), and ending work in...
-
Joe Schmaltz has carried on a retail business for about 20 years. He intends to transfer the business assets and liabilities to a corporation, Schmaltz Enterprises Ltd. (SEL), in which he will own...
-
4. (5 points) Consider the following function: f(x) = 5x3 + 7x+3. a. (3 points) Determine the equation of the tangent line to f(x) at x=1. b. (1 point) Use your result from part a to approximate...
-
XYZ Co. will require 2 million Polish zloty in 3 years to purchase imports. Assume interest rate parity holds. Assume that the spot rate of the Polish zloty is $.30. The 3-year annualized interest...
-
What unique concerns face an auditor when a service center is being used to provide EDP support?
-
Describe the common computer audit techniques available to systems testing. Elaborate on their relative advantages and disadvantages.
-
Describe the capabilities of generalized audit software.
-
What are the major phases of an EDP audit program?
-
List three input, processing, and output controls.
-
The managers of a firm should not have a notion that the retained re "free" because the cost of retained earnings is equal to the cost of thout flotation costs
-
Anne is employed by Bradley Contracting Company. Bradley has a $1.3 million contract to build a small group of outbuildings in a national park. Anne alleges that Bradley Contracting has discriminated...
-
A bank in California has 13 branches spread throughout northern California, each with its own minicomputer where its data are stored. Another bank has 10 branches spread through- out California, with...
-
Discuss why any distinction between IS auditing and financial auditing is not meaningful.
-
Discuss the key features of Section 404 of the Sarbanes-Oxley Act.

Study smarter with the SolutionInn App